Getting Started with Python for Data Analysis
Data analysis is a critical skill in today's data-driven world. Python, with its powerful libraries and simple syntax, has become the go-to language for data analysts and scientists. This blog will guide you through the essentials of getting started with Python for data analysis, providing you with the tools and knowledge to begin your journey.
1. Why Python for Data Analysis?
Python is popular for data analysis due to its simplicity, readability, and extensive ecosystem of libraries. It offers several advantages:
- Ease of Learning: Python's syntax is straightforward and readable, making it accessible for beginners.
- Comprehensive Libraries: Libraries like Pandas, NumPy, Matplotlib, and Seaborn provide robust tools for data manipulation, analysis, and visualization.
- Community Support: A large community means extensive documentation, tutorials, and forums to help you overcome challenges.
2. Setting Up Your Environment
Before diving into data analysis, you need to set up your Python environment. Here’s how:
Installing Python
Download and install Python from the official website. Ensure you add Python to your system's PATH during installation.
Installing Jupyter Notebook
Jupyter Notebook is an open-source web application that allows you to create and share documents containing live code, equations, visualizations, and narrative text. Install Jupyter Notebook using pip:
pip install jupyter
To start Jupyter Notebook, simply run:
jupyter notebook
This command will open a new tab in your default web browser with the Jupyter interface.

Installing Essential Libraries
Install the following libraries using pip:
pip install numpy pandas matplotlib seaborn
3. Introduction to Key Libraries
NumPy
NumPy is the fundamental package for scientific computing with Python. It provides support for arrays, matrices, and many mathematical functions.
import numpy as np
# Create an array
data = np.array([1, 2, 3, 4, 5])
print(data)
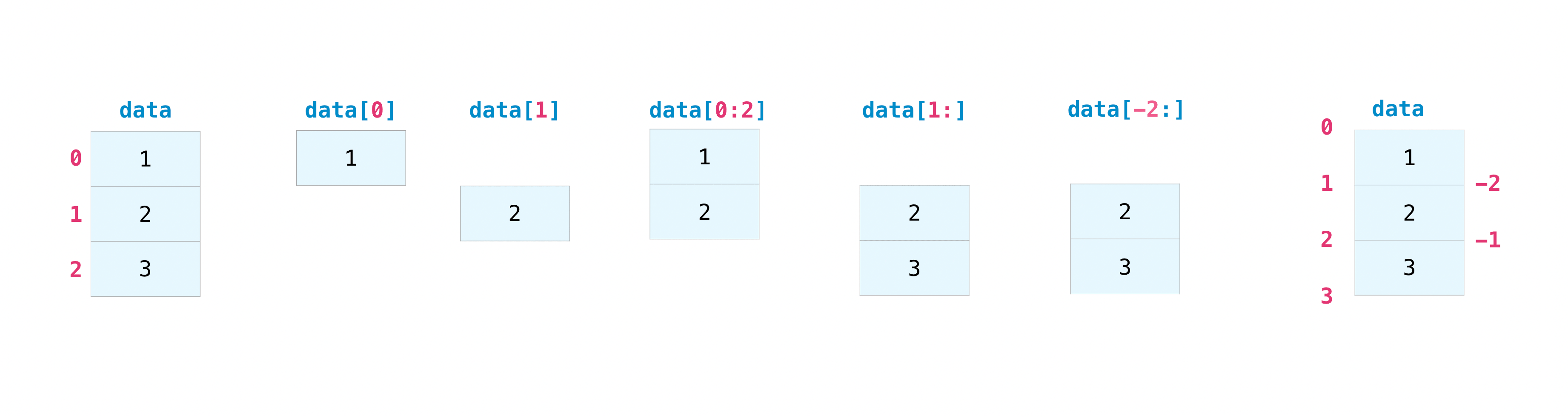
Pandas
Pandas is a powerful data manipulation tool that provides data structures like Series and DataFrame.
import pandas as pd
# Create a DataFrame
data = {'Name': ['John', 'Anna', 'Peter', 'Linda'],
'Age': [28, 24, 35, 32]}
df = pd.DataFrame(data)
print(df)
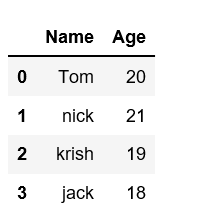
Matplotlib and Seaborn
Matplotlib is a plotting library for creating static, animated, and interactive visualizations. Seaborn builds on Matplotlib and provides a high-level interface for drawing attractive statistical graphics.
import matplotlib.pyplot as plt
import seaborn as sns
# Create a simple plot
x = [1, 2, 3, 4, 5]
y = [10, 15, 13, 17, 19]
plt.plot(x, y)
plt.title('Simple Plot')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()

# Create a Seaborn plot
sns.set(style="darkgrid")
tips = sns.load_dataset("tips")
sns.relplot(x="total_bill", y="tip", hue="smoker", data=tips)
plt.show()

4. Loading and Inspecting Data
Pandas makes it easy to load and inspect data. You can read data from various formats like CSV, Excel, and SQL databases.
# Load a CSV file
df = pd.read_csv('data.csv')
# Display the first few rows
print(df.head())
# Get a summary of the DataFrame
print(df.info())
5. Data Cleaning and Preparation
Data often needs to be cleaned and prepared before analysis. This includes handling missing values, removing duplicates, and transforming data.
Handling Missing Values
# Check for missing values
print(df.isnull().sum())
# Fill missing values
df.fillna(method='ffill', inplace=True)
Removing Duplicates
# Remove duplicate rows
df.drop_duplicates(inplace=True)
Transforming Data
# Convert data types
df['column'] = df['column'].astype('int')
6. Exploratory Data Analysis (EDA)
EDA involves summarizing the main characteristics of a dataset, often using visual methods.
Descriptive Statistics
# Summary statistics
print(df.describe())
Data Visualization
# Histogram
df['column'].hist()
plt.show()
# Scatter plot
plt.scatter(df['column1'], df['column2'])
plt.show()
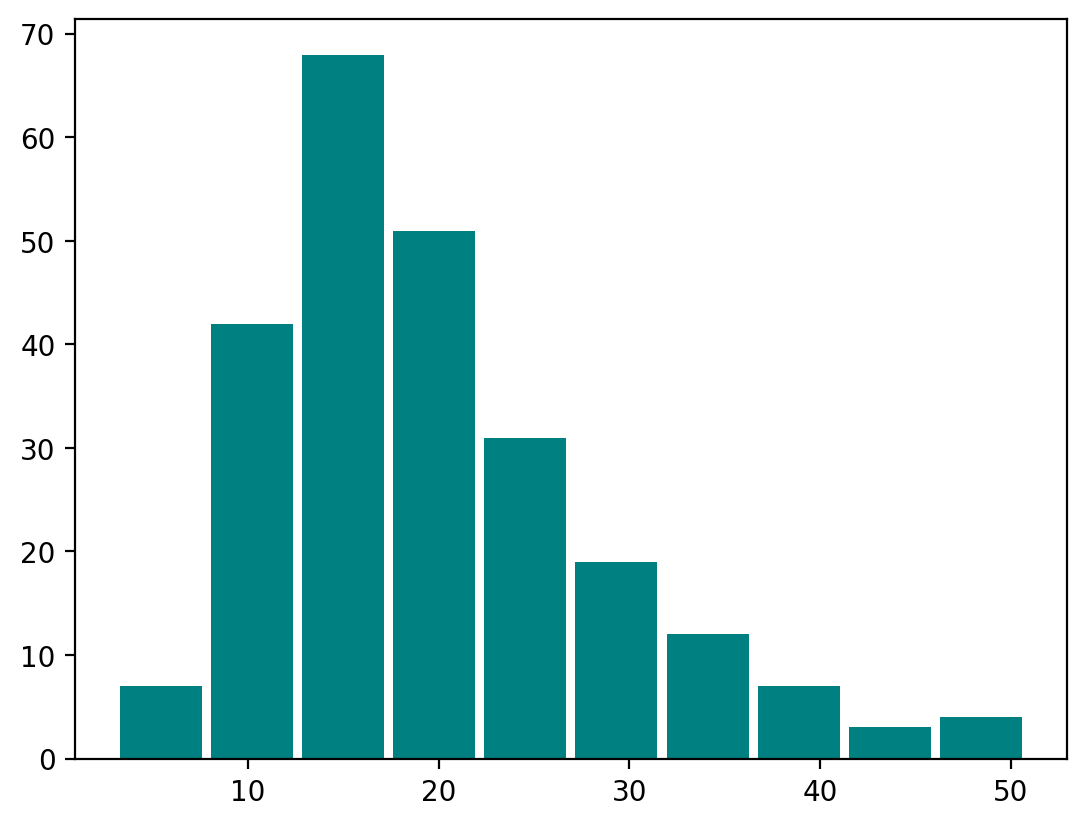

7. Conclusion
Getting started with Python for data analysis involves setting up your environment, understanding key libraries, and learning basic data manipulation and visualization techniques. As you gain more experience, you can explore advanced topics like machine learning, deep learning, and big data processing.
References
- Python Official Website
- NumPy Documentation
- Pandas Documentation
- Matplotlib Documentation
- Seaborn Documentation
IMAGES ADDED ARE JUST EXAPMPLES AND REFERRED FROM INTERNET
Best Practices for Data Cleaning and Preprocessing
Data cleaning and preprocessing are essential steps in the data science pipeline. These processes ensure that your data is accurate, consistent, and ready for analysis. In this blog post, we will explore best practices for data cleaning and preprocessing to help you achieve high-quality, reliable datasets.
1. Understand Your Data
Before diving into cleaning and preprocessing, it's crucial to understand the data you're working with. This includes knowing:
- The source of the data
- The structure of the data
- The meaning of each feature (column)
- The type of data (categorical, numerical, etc.)
2. Handle Missing Values
Missing data can skew your analysis and lead to inaccurate conclusions. Here are a few strategies for handling missing values:
- Remove Missing Values: If the dataset is large and the number of missing values is small.
- Impute Missing Values: Use statistical methods (mean, median, mode) or predictive models to fill in missing values.
- Flag and Fill: Create a new column indicating the presence of missing values and fill them with a placeholder.

3. Remove Duplicates
Duplicate data can distort your analysis. Ensure to:
- Identify duplicates using key columns.
- Remove exact duplicates or decide on a method for handling near-duplicates based on the context.
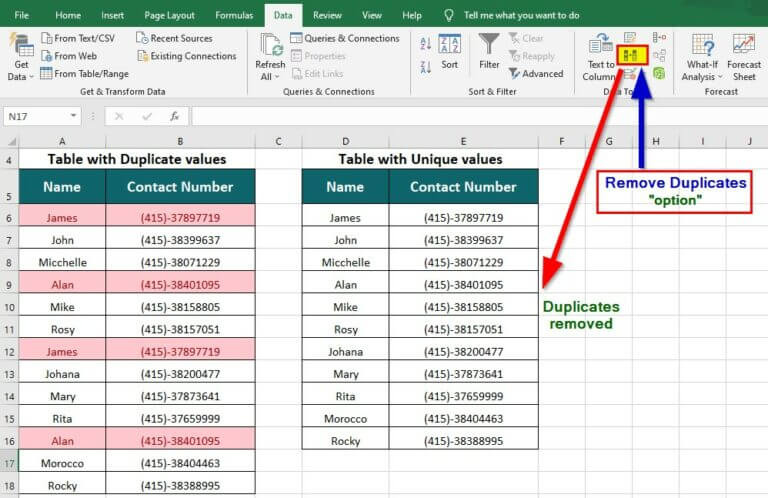
4. Handle Outliers
Outliers can significantly impact the results of your analysis. Depending on the context:
- Remove Outliers: If they are errors or irrelevant.
- Cap or Transform Outliers: If they are valid but extreme, consider capping values at a certain percentile or transforming the data using techniques like log transformation.

A box plot showing the identification of outliers in a dataset.
5. Standardize and Normalize Data
Ensuring that your data is on a comparable scale is vital, especially for algorithms that are sensitive to the scale of input features.
- Normalization: Rescale the data to have a mean of 0 and a standard deviation of 1.
- Standardization: Scale data to a [0, 1] range.
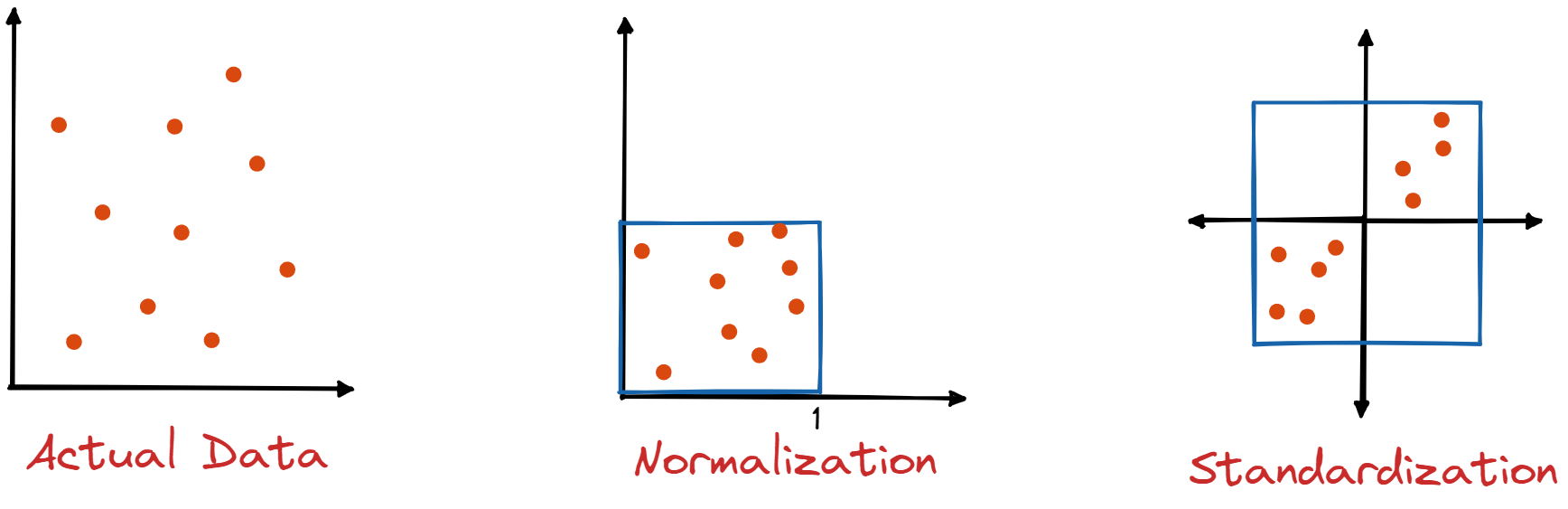
Comparison of raw data, standardized data, and normalized data on a graph.
6. Encode Categorical Variables
Many machine learning algorithms require numerical input. Convert categorical variables into numerical format using:
- Label Encoding: Assign a unique number to each category.
- One-Hot Encoding: Create binary columns for each category.

Example of categorical data before and after label and one-hot encoding.
7. Feature Engineering
Creating new features from existing ones can improve model performance. Examples include:
- Date Features: Extracting day, month, year, or creating time-based features.
- Interaction Features: Combining features to capture interactions.
8. Data Splitting
Split your data into training, validation, and test sets to ensure your model's generalizability.
- Training Set: Used to train the model.
- Validation Set: Used to tune the model and prevent overfitting.
- Test Set: Used to evaluate the final model performance.
Visualization of a dataset being split into training, validation, and test sets.
Conclusion
Data cleaning and preprocessing are critical steps in the data science process. By following these best practices, you can ensure that your data is accurate, consistent, and ready for analysis, leading to more reliable and valid results.